Moving platforms vertically and horizontally in GMS2 can be tricky, but I feel it is a definitely must-have item if you are designing a platformer title. Below is my process for creating moving jump-thru platforms.
The Parent
Since we are going to have vertical/horizontal platforms, I usually create a parent/child system to organize them better and inherit qualities.
object: oJumpThroughPlatform
//Parent Object: oJumpThroughPlatform
//Create Event
_vspd = 0;
_hspd = 0;
_platDir = choose(-1,1);
//EndStep Event
switch (object_index)
{
//Horizontal Platform
case oHMovingPlatform:
_hspd = _platDir * _moveSpeed;
var wallCollision = place_meeting(x+_hspd, y, oPlatformStop);
if (wallCollision)
{
_platDir *= -1;
}
//Adjust the speed of the player
//Player object will inherit the speed of the platform they are colliding with
with (oPlayer)
{
if (!place_meeting(x +other._hspd, y, oPlatformStop)) {
if (place_meeting(x, y + 1, other) && !place_meeting(x, y, other)) {
x+=other._hspd;
}
}
}
x += _hspd;
break;
//Vertical Platform
case oVMovingPlatform:
_vspd = _platDir * _moveSpeed;
var heightOffset = 24;
var upAndDownWallCollisions = place_meeting(x, y + _vspd+(heightOffset*_platDir), oPlatformStop);
if (upAndDownWallCollisions) {
_platDir *= -1;
}
//Adjust the speed of the player
//Player object will inherit the speed of the platform they are colliding with
#region MovePlayer
with(oPlayer)
{
if (!place_meeting(x , y+other._vspd, oPlatformStop)) {
if(place_meeting(x, y + abs(other._vspd), other) && !place_meeting(x, y, other))
{
y += other._vspd;
}
}
}
#endregion
y += _vspd;
break;
}
Additionally, I add a couple a _moveSpeed Variable Definitions to this object so that I am able to manipulate speeds in through the level editor.

Platform Stop
You’ll notice that collision for these platforms is managed with an object name oPlatformStop. This is an empty object, it requires no code at all. Simple place it in the level editor at the start and end points you would like for the moving platform. You could have an xStart/xEnd, yStart/yEnd and use variable definitions and actually screen coordinates. I’ve tried those, but since moving to the oPlatformStop object it has been much better and quicker to iterate levels.
Below is a quick screen shot of the level editor illustrating the use of the oPlatformStop objects to control the movement of the platforms.
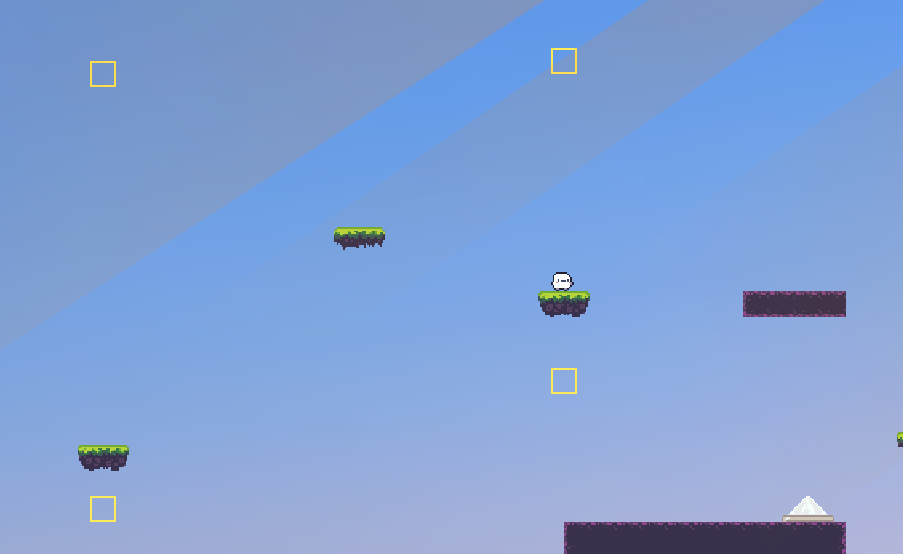
Child Objects
I make two additional Objects for Horizontal/Vertical platforms. I reference these in the above code in the Parent Object End Step, so make sure these are referenced properly. They both will inherit from the Create and End Step, no code is really required for these moving platforms. This is an elegant approach as all the code is performed in one object.